Projekt
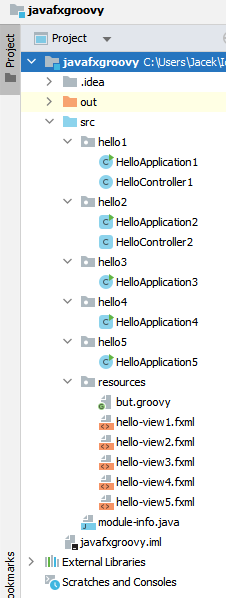
Pakiet | Aplikacja | Kontroler |
---|---|---|
hello1 | Java | Java |
hello2 | Groovy | Groovy |
hello3 | Java | skrypt Groovy wbudowany |
hello4 | Groovy | skrypt Groovy wbudowany |
hello5 | Java | skrypt Groovy zewnętrzny |
Klasy
HelloApplication1.java
package hello1; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Scene; import javafx.stage.Stage; public class HelloApplication1 extends Application { public static void main(String[] args) { Application.launch(args); } @Override public void start(Stage stage) { try{ FXMLLoader fxmlLoader = new FXMLLoader(HelloApplication1.class.getResource("/resources/hello-view1.fxml")); Scene scene = new Scene(fxmlLoader.load(), 320, 240); stage.setTitle("Hello!"); stage.setScene(scene); stage.show(); } catch (Exception e) { e.printStackTrace(); } } }
HelloController1.java
package hello1; import javafx.fxml.FXML; import javafx.scene.control.Label; public class HelloController1 { public HelloController1(){} @FXML private Label welcomeText; @FXML private void onHelloButtonClick() { welcomeText.setText("Welcome to JavaFX Application!"); } }
HelloApplication2.groovy
package hello2 import groovy.transform.CompileStatic import javafx.application.Application import javafx.fxml.FXMLLoader import javafx.scene.Parent import javafx.scene.Scene import javafx.stage.Stage @CompileStatic class HelloApplication2 extends Application { @Override void start(Stage stage) { def fxmlLoader = new FXMLLoader(HelloApplication2.class.getResource("/resources/hello-view2.fxml")) def scene = new Scene(fxmlLoader.load() as Parent, 320, 240) stage.setTitle("Hello!") stage.setScene(scene) stage.show() } static void main(String[] args) { launch(HelloApplication2) } }
HelloController2.groovy
package hello2 import javafx.fxml.FXML import javafx.scene.control.Label class HelloController2 { @FXML private Label welcomeText @FXML protected void onHelloButtonClick() { welcomeText.setText("Welcome to JavaFX Application!") } }
HelloApplication3.java
package hello3; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Scene; import javafx.stage.Stage; public class HelloApplication3 extends Application { public static void main(String[] args) { Application.launch(args); } @Override public void start(Stage stage) { try{ FXMLLoader fxmlLoader = new FXMLLoader(HelloApplication3.class.getResource("/resources/hello-view3.fxml")); Scene scene = new Scene(fxmlLoader.load(), 320, 240); stage.setTitle("Hello!"); stage.setScene(scene); stage.show(); } catch (Exception e) { e.printStackTrace(); } } }
HelloApplication4.groovy
package hello4 import groovy.transform.CompileStatic import javafx.application.Application import javafx.fxml.FXMLLoader import javafx.scene.Parent import javafx.scene.Scene import javafx.stage.Stage @CompileStatic class HelloApplication4 extends Application { @Override void start(Stage stage) { def fxmlLoader = new FXMLLoader(HelloApplication4.class.getResource("/resources/hello-view4.fxml")) def scene = new Scene(fxmlLoader.load() as Parent, 320, 240) stage.setTitle("Hello!") stage.setScene(scene) stage.show() } static void main(String[] args) { launch(HelloApplication4) } }
HelloApplication5.java
package hello5; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Scene; import javafx.stage.Stage; public class HelloApplication5 extends Application { public static void main(String[] args) { Application.launch(args); } @Override public void start(Stage stage) { try{ FXMLLoader fxmlLoader = new FXMLLoader(HelloApplication5.class.getResource("/resources/hello-view5.fxml")); Scene scene = new Scene(fxmlLoader.load(), 320, 240); stage.setTitle("Hello!"); stage.setScene(scene); stage.show(); } catch (Exception e) { e.printStackTrace(); } } }
module-info.java
module javafxgroovy { requires java.sql; requires javafx.graphics; requires javafx.controls; requires javafx.fxml; requires org.apache.groovy; requires org.apache.groovy.sql; opens hello1 to javafx.fxml; opens hello2 to javafx.fxml; opens hello3 to javafx.fxml; opens hello4 to javafx.fxml; opens hello5 to javafx.fxml; exports hello1 to javafx.graphics, javafx.fxml; exports hello2 to javafx.graphics, javafx.fxml; exports hello3 to javafx.graphics, javafx.fxml; exports hello4 to javafx.graphics, javafx.fxml; exports hello5 to javafx.graphics, javafx.fxml; }
hello-view1.fxml
<?xml version="1.0" encoding="UTF-8"?> <?import javafx.geometry.Insets?> <?import javafx.scene.control.Label?> <?import javafx.scene.layout.VBox?> <?import javafx.scene.control.Button?> <VBox alignment="CENTER" spacing="20.0" xmlns:fx="http://javafx.com/fxml" fx:controller="hello1.HelloController1"> <padding> <Insets bottom="20.0" left="20.0" right="20.0" top="20.0"/> </padding> <Label fx:id="welcomeText"/> <Button text="Hello!" onAction="#onHelloButtonClick"/> </VBox>
hello-view2.fxml
<?xml version="1.0" encoding="UTF-8"?> <?import javafx.geometry.Insets?> <?import javafx.scene.control.Label?> <?import javafx.scene.layout.VBox?> <?import javafx.scene.control.Button?> <VBox alignment="CENTER" spacing="20.0" xmlns:fx="http://javafx.com/fxml" fx:controller="hello2.HelloController2"> <padding> <Insets bottom="20.0" left="20.0" right="20.0" top="20.0"/> </padding> <Label fx:id="welcomeText"/> <Button text="Hello!" onAction="#onHelloButtonClick"/> </VBox>
hello-view3.fxml
<?xml version="1.0" encoding="UTF-8"?> <?language groovy?> <?import javafx.geometry.Insets?> <?import javafx.scene.control.Label?> <?import javafx.scene.layout.VBox?> <?import javafx.scene.control.Button?> <VBox alignment="CENTER" spacing="20.0" xmlns:fx="http://javafx.com/fxml"> <padding> <Insets bottom="20.0" left="20.0" right="20.0" top="20.0"/> </padding> <Label fx:id="welcomeText"/> <Button text="Hello!" onAction="onHelloButtonClick()"/> <fx:script> def onHelloButtonClick() { welcomeText.setText("Welcome to JavaFX Application!") } </fx:script> </VBox>
hello-view4.fxml
<?xml version="1.0" encoding="UTF-8"?> <?language groovy?> <?import javafx.geometry.Insets?> <?import javafx.scene.control.Label?> <?import javafx.scene.layout.VBox?> <?import javafx.scene.control.Button?> <VBox alignment="CENTER" spacing="20.0" xmlns:fx="http://javafx.com/fxml"> <padding> <Insets bottom="20.0" left="20.0" right="20.0" top="20.0"/> </padding> <Label fx:id="welcomeText"/> <Button text="Hello!" onAction="onHelloButtonClick()"/> <fx:script> def onHelloButtonClick() { welcomeText.setText("Welcome to JavaFX Application!") } </fx:script> </VBox>
hello-view5.fxml
<?xml version="1.0" encoding="UTF-8"?> <?language groovy?> <?compile true?> <?import javafx.geometry.Insets?> <?import javafx.scene.control.Label?> <?import javafx.scene.layout.VBox?> <?import javafx.scene.control.Button?> <VBox alignment="CENTER" spacing="20.0" xmlns:fx="http://javafx.com/fxml"> <padding> <Insets bottom="20.0" left="20.0" right="20.0" top="20.0"/> </padding> <Label fx:id="welcomeText"/> <Button text="Hello!" onAction="onHelloButtonClick()"/> <fx:script source="but.groovy" /> </VBox>
but.groovy
package resources def welcomeText def onHelloButtonClick() { welcomeText.setText("Welcome to JavaFX Application!") }
Wnioski
W aplikacjach JavaFX, Java może być swobodnie zastępowana przez Groovy. Zarówno Java jak i Groovy mogą rozszerzać klasy JavaFX. Skrypty Groovy mogą być używane w plikach FXML i są doskonałym zamiennikiem wycofanych skryptów Nashorn.