JavaFX: prosty stoper cyfrowy
Klasa Listing14c_03
Ściągnij klasę Listing14c_03
package rozdzial14c; import javafx.animation.AnimationTimer; import javafx.application.Application; import javafx.application.Platform; import javafx.beans.property.BooleanProperty; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.ToggleButton; import javafx.scene.layout.*; import javafx.scene.paint.Color; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.stage.Stage; import java.time.Instant; import java.time.Duration; public class Listing14c_03 extends Application { private static final Font CLOCK_FONT = Font.font("Arial", FontWeight.BOLD, 20); private static final Background CLOCK_BACKGROUND = new Background(new BackgroundFill(Color.HONEYDEW, CornerRadii.EMPTY, new Insets(0, 0, 0, 0))); private Label label; private ToggleButton tbutton; private AnimationTimer timer; public static void main(String[] args) {Application.launch(args);} @Override public void start(Stage stage) { try { VBox root = new VBox(); label = new Label(); label.setText("00:00:00"); label.setFont(CLOCK_FONT); label.setTextFill(Color.GREEN); timer = new AriaTimer(); tbutton = new ToggleButton("Start"); BooleanProperty sel = tbutton.selectedProperty(); sel.addListener((observable, oldValue, newValue) -> { tbutton.setText(newValue ? "Stop" : "Start"); if (newValue) { timer = new AriaTimer(); timer.start(); } else { timer.stop(); } }); root.setBackground(CLOCK_BACKGROUND); root.getChildren().addAll(label, tbutton); Scene scene = new Scene(root, 200, 50); stage.setScene(scene); stage.setTitle("Stoper Cyfrowy"); stage.setOnCloseRequest(event -> Platform.exit()); stage.show(); } catch (Exception e) { e.printStackTrace(); } } private class AriaTimer extends AnimationTimer { final Instant start = Instant.now(); @Override public void handle(long now) { Instant end = Instant.now(); long millis = (Duration.between(start, end)).toMillis(); long second = (millis / 1000) % 60; long milli = millis - second * 1000; long minute = (millis / (60000)) % 60; long hour = millis / (3600000); String time = String.format("%02d:%02d:%02d.%d", hour, minute, second, milli); label.textProperty().set(time); } } }
Po uruchomieniu klasy, uruchomieniu i zatrzymaniu stopera zobaczymy (Rys. 14_04)
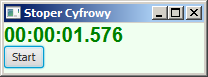